C# Main() Method
Introduction to C# Main() Method
किसी भी C# application में Main() method वह entry point होता है जँहा से program का execution शुरू होता है। जब application start होती है या program को run किया जाता है तो Main() method वह पहला method होता है जो call होता है।
C# console application और windows forms (Desktop) applications में Main() method define किया जाना आवश्यक होता है। लेकिन C# libraries और service applications में Main() method की entry point के रूप में आवश्यकता नहीं होती है।
एक C# program में Main() method कई हो सकते है लेकिन entry point केवल एक ही हो सकता है। यदि आपके program में एक से अधिक classes में Main() method define किया गया है तो ऐसे में आपको program को /main compiler option द्वारा compile करना होगा। इस option द्वारा बताया जाता है की आप किस Main() method को entry point के रूप में use करेंगे।
इस option का प्रयोग करने के लिए आप program को run करवाते समय उसके नाम के आगे /main लिखकर उसके बाद colon लगाकर उस class का नाम लिखते है जिसके main method को आप entry point के रूप में use करना चाहते है। इसका syntax निचे दिया जा रहा है।
csc myprogram.cs /main:class-name
लेकिन इस प्रकार आप सिर्फ command line compiler में ही program run करवा सकते है। यदि आप Visual Studio Express use कर रहे है तो इसके लिए आपको Project menu से निचे ही निचे दिया गया आपके project की properties का option choose करना होगा।
इसके बाद आप application tab पर click करेंगे। इसके बाद आप Startup object option से अपना entry point choose कर सकते है। जैसा की निचे दी गयी image में show हो रहा है।
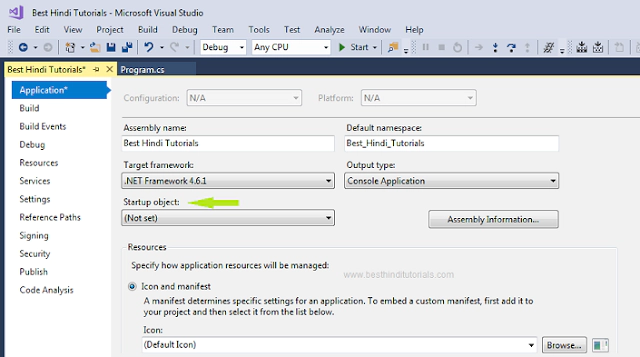
आपको एक बात ध्यान रखनी चाहिए की C# में Main() method किसी class के अलावा struct के अंदर भी define किया जाता है। आइये अब Main() method के syntax को समझने का प्रयास करते है।
Syntax of C# Main() Method
C# Main() method का general syntax निचे दिया जा रहा है।
static return-type Main(string[] args)
{
//code here...
}
जैसा की आप ऊपर दिए गए syntax में देख सकते है Main() method का syntax बहुत ही simple है। इसमें कुछ keywords है जिनके बारे में निचे detail से समझाया जा रहा है।
static
C# में Main() method को static define किया जाना आवश्यक होता है। Static members को बिना class का object create किये access किया जा सकता है। जैसा की आपको पता है Main() method program का entry point होता है इसलिए इसे static define किया जाता है ताकि program की शुरआत में इसे बिना कोई object create किये call किया जा सके।
return-type
C# में Main() method का return type आप void या int define कर सकते है। जब आप Main method को void define करते है तो Main() method के अंदर आपको किसी प्रकार की value return करने की आवश्यकता नहीं होती है। लेकिन void define किये जाने पर भी background में यह method 0 return करता है।
जब आप Main() method के लिए return type int define करते है तो आपको program में integer value return करवानी होती है। यदि आप कोई value return नहीं करवाते है तो Main() method के आखिर में return 0 statement लिखते है।
Main
C# में जब आप Main() method define करते है तो यह आवश्यक है की इसका पहला अक्षर (M) capital में लिखा जाए। यदि आप ऐसा नहीं करते है तो error generate होती है।
Command Line Arguments
Main() method के brackets में define किये गए string[] args को command line arguments कहा जाता है। C# में Main() method की standard declaration में command line arguments define किये जाते है। हालाँकि आप command line arguments के बिना भी Main() को define कर सकते है लेकिन यह standard तरीका नहीं होगा और इसे recommend नहीं किया जाता है।
Command line arguments के द्वारा आप Main() method को arguments भेज सकते है। उन arguments को Main() method में किसी प्रकार की processing के लिए use किया जा सकता है।
उदाहरण के लिए आप command line arguments को किसी command के रूप में use कर सकते है और अलग अलग command दिए जाने पर अलग अलग tasks perform कर सकते है।
Command line arguments असल में एक String array है जो आपके द्वारा pass किये गए arguments को store करता है। इन arguments को Convert class द्वारा आप numeric में भी convert कर सकते है।
Command line arguments program को run करते समय pass किये जाते है। Command line compiler के case में इन arguments को program के नाम के बाद space लगाकर लिखा जाता है। इसका syntax निचे दिया जा रहा है।
csc myProgram.cs // for creating .exe file
myProgram cArg1 cArg2 ... cArgN // program name then argument separated with space.
यदि आप Visual Studio use कर रहे है तो command line arguments define करने के लिए सबसे पहले आपको Project menu पर click करना होगा इसके बाद आप उस menu से निचे ही निचे दिया गया Properties option choose करेंगे।
इसके बाद Properties का box open होगा। इस box में left side से आप debug option को choose करेंगे। इसके बाद आपको right side में Command line arguments का option show होगा जिसमे आप command line arguments define कर सकते है।
निचे command line arguments को एक simple उदाहरण द्वारा समझाया जा रहा है। इस उदाहरण में command line argument के रूप में pass किये गए user के नाम को Hello message के साथ output के रूप में show किया जाएगा।
using System;
class claDemo
{
static void Main(String[] args)
{
Console.WriteLine("Hello {0}",args[0]);
Console.ReadKey();
}
}
ऊपर दिए गए उदाहरण को run करते समय आपको कोई नाम command line argument के रूप में pass करना होगा।आपको output show करने के लिए इसमें Reader pass किया गया है। यह उदाहरण निचे दिया गया output generate करता है।
Hello Reader