PHP Constructor & Destructor
Introduction to PHP Constructor
Constructor एक function होता है जो class के अंदर declare किया जाता है। जब भी class का कोई नया object create किया जाता है तो class constructor को automatically call करती है। Constructor उन situations के लिए महत्वपूर्ण होता है जब object को use करने से पहले आप कोई intialization perform करना चाहते है जैसे की class member variables को values assign करना, database से connection establish करना आदि।
PHP 5 आपको special constructor function declare करने की सुविधा प्रदान करती है। PHP के पुराने version (PHP 4) में constructor class के नाम जैसा function create करके declare किया जाता था। लेकिन PHP 5 आपको इसके लिए special function __construct() provide करती है।
PHP 5 को पुराने version से compatible बनाने के लिए ये छूट दी गयी है की आप दोनों में से किसी भी तरीके से constructor declare कर सकते है। जब भी कोई object create होगा तो PHP सबसे पहले __construct() function को search करके execute करने का प्रयास करेगी। यदि PHP को __construct function नहीं मिलता है तो वह class के नाम वाले function को ढूंढेगी और उसे execute करने का प्रयास करेगी।
Syntax of PHP Constructor Function
PHP में __construct() function declare करने का general syntax निचे दिया जा रहा है।
function __construct($args-list)
{
//Statements to be executed when objects are created.
}
किसी भी normal function की तरह constructor function declare करने के लिए आप function keyword use करते है। इसके बाद double underscore लगाकर __construct() function का नाम लिखते है। Constructor function में आप arguments भी pass कर सकते है।
Examples of PHP Constructor
PHP में constructor create करने का simple उदाहरण निचे दिया जा रहा है।
<?php
class myClass
{
function __construct()
{
echo "Object is created...";
}
}
$myclass = new myClass;
?>
ऊपर दिए गए उदाहरण में जैसे ही myClass का object create किया जाता है तो constructor function call होता है और Object is created… message display होता है।
जैसा की मैने आपको बताया किसी भी normal function की तरह contructor function में भी arguments pass किये जा सकते है। इसका उदाहरण निचे दिया जा रहा है।
<?php
class myClass
{
function __construct($num)
{
echo "Object number $num is created";
}
}
$myclass = new myClass(5);
?>
ऊपर दिया गया उदाहरण निचे दिया गया output generate करता है।
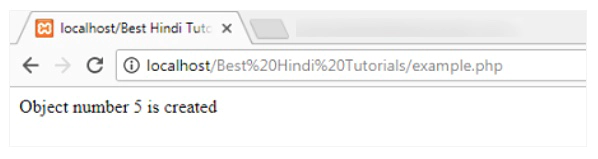
PHP Constructor and Inheritance
यदि child class में constructor function define किया गया है तो parent class का constructor नहीं call किया जाएगा। यदि आप parent constructor को भी call करना चाहते है तो इसके लिए आपको child class के constructor में parent class के constructor को इस प्रकार call करना होगा।
parent::__construct()
यदि child class कोई constructor define नहीं करती है तो implicitly parent class का constructor call किया जाता है। लेकिन ऐसा तब ही होता है जब parent class में constructor को private नहीं declare किया गया हो।
Introduction to PHP Destructor
Destructor एक special function होता है। जिस प्रकार constructor function object create करने पर call होता है उसी प्रकार destructor function object destroy होने पर call होता है।
कुछ programming languages में objects को manually destroy करना होता है, लेकिन PHP में यह काम garbage collector द्वारा किया जाता है। जैसे ही कोई object free होता है और उसकी अतिरिक्त आवश्यकता नहीं होती है तो garbage collector उसे destroy कर देता है।
Syntax of PHP Destructor
PHP में destructor __destruct() function द्वारा declare किया जाता है। इसका general syntax निचे दिया जा रहा है।
function __destruct()
{
//Statements to be executed when object gets destroyed.
}
Constructor function की ही तरह destructor function भी double underscore function द्वारा define किया जाता है।
Example of PHP Destructor
PHP destructor function का उदाहरण निचे दिया जा रहा है।
<?php
class myClass
{
function __construct()
{
echo "Object is created...<br />";
}
function __destruct()
{
echo "Object is destroyed";
}
}
$myclass = new myClass;
?>
ऊपर दिए उदाहरण में object create होने पर object is created message और object destroy होने पर object is destroyed message display होगा।